How I Secure My Laravel + React Apps as a Beginner Developer (Real-World Guide)
Published on by Anas Ahson
As a beginner web developer, I used to overlook security. I assumed "Laravel handles most of it" or "React is just frontend — no big deal."
That changed when my first full-stack app got hit with fake signups and odd requests. Security isn’t optional.
Securing Laravel: Lessons from My Mistakes
1. Laravel Sanctum for Easy API Authentication
Connecting React to Laravel APIs was a mess until I found Laravel Sanctum. I struggled with token management, CSRF errors, and unprotected routes.
composer require laravel/sanctum
In routes/api.php
:
Route::middleware('auth:sanctum')->get('/user', function (Request $request) {
return $request->user();
});
Tip: Test routes with and without tokens. I once skipped middleware, and my endpoint was wide open.
2. Composer Security Checker
I used an outdated package with a vulnerability — unknowingly. Now, I run this before deploying:
composer require enlightn/security-checker --dev
php artisan security:check
Lesson: Outdated packages can silently compromise your app.
3. Debug Mode in Production
I shipped a project with APP_DEBUG=true
, exposing stack traces publicly. Now, I ensure APP_DEBUG=false
before every deployment.
Securing React: Client-Side Isn’t Safe Either
1. DOMPurify Against XSS Attacks
My React comment box used dangerouslySetInnerHTML
. Someone tested this:
<img src=x onerror=alert('XSS-attack!')>
I now sanitize inputs with:
import DOMPurify from 'dompurify';
const cleanHTML = DOMPurify.sanitize(userInput);
Takeaway: Never trust user input, even from logged-in users.
2. Axios with CSRF and Tokens
CSRF errors with Laravel Sanctum were brutal. Here’s the fix:
axios.get('/sanctum/csrf-cookie').then(() => {
axios.post('/login', credentials).then(...);
});
axios.defaults.withCredentials = true;
Mistake: Forgetting withCredentials
cost me an hour of debugging 401 errors.
My Full-Stack Security Habits
1. Laravel Telescope for Monitoring
In dev mode, Telescope tracks API requests, auth attempts, and failed logins:
composer require laravel/telescope --dev
php artisan telescope:install
It caught someone brute-forcing tokens on my API.
2. Deployment Security Checklist
- ✅ HTTPS enabled
- ✅
APP_DEBUG=false
- ✅
.env
in.gitignore
- ✅ Run
php artisan security:check
- ✅ DOMPurify on React inputs
- ✅ Axios with
withCredentials
- ✅ Check headers on securityheaders.com
Final Thoughts: Security is a Journey
Security isn’t a one-time fix — it’s a skill built over time. Test like a hacker, patch what breaks, and repeat.
Want the codebase? Let me know, and I’ll share it on GitHub!
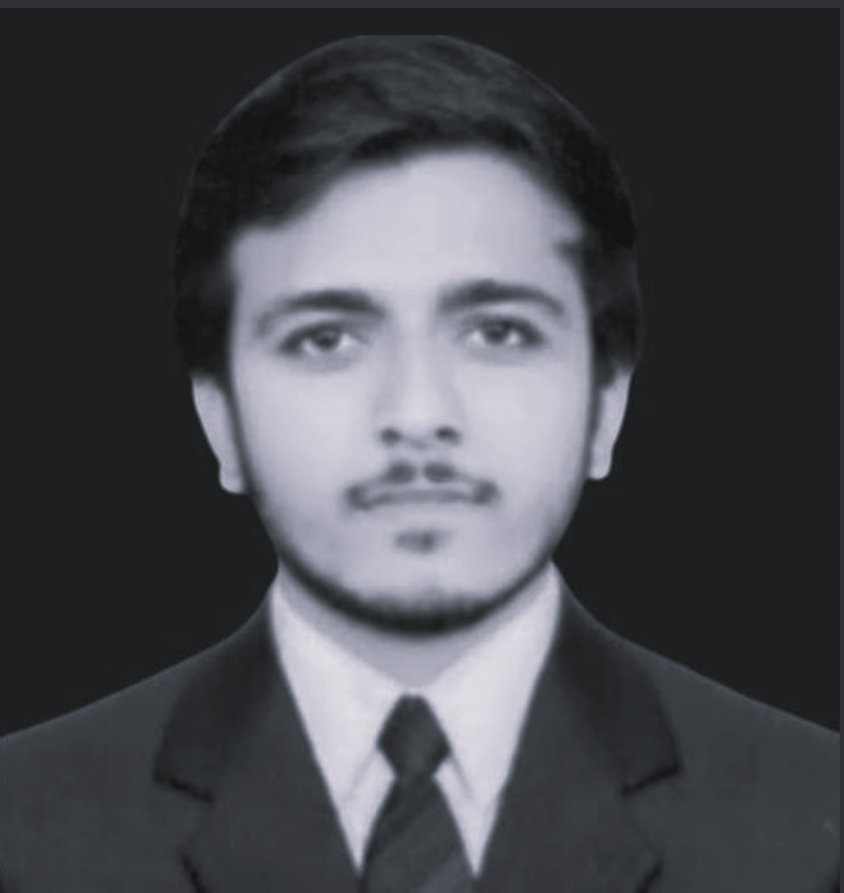
About Anas Ahson
Anas Ahson is a Full Stack Developer specializing in Laravel and PHP, passionate about building efficient web solutions and sharing knowledge through articles like this one.
More about the author →